WhatsApp Flow
Handle & process flow templates
WhatsApp introduced flows, mobile-app-screen like message types.
This type makes collecting user information a breeze and greatly improves your chatbot UX
WCE has out-of-the box support for flows. An example flow template will be as below
"CURRENT-STAGE-NAME":
kind: flow
message:
flow-id: flow-id
draft: true
name: flow-name
title: Flow Title
body: Flow message body
button: flow-cta-button
routes:
"re:.*": "NEXT_STAGE"
WCE tries to keep template signature similar, a flow template can have a title
, body
and or footer
like any other interactive message template.
Dynamic properties - Passing initial data
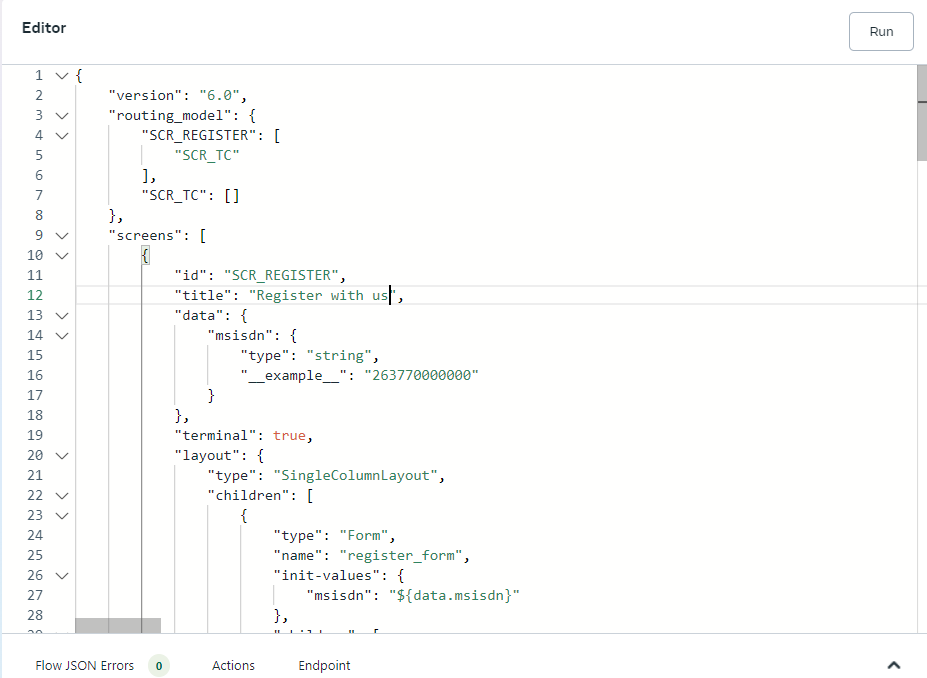
You may have a flow that requires initial values (Dynamic Properties). Use cases for this can be if you have a flow that takes in initial dropdown items or just to populate a field with a default value.
The template "template"
hook can be used for this.
"STAGE-NAME":
kind: flow
template: "example.hooks.flows.get_register_defaults"
on-receive: "example.hooks.flows.register"
message:
flow-id: 1234567890
draft: true
name: SCR_REGISTER
title: "New Account"
body: "Register with us to access our services"
button: Register
routes:
"re:.*": "STAGE-USER-LOOKUP"
You have to define a template as below
# example/hooks/flows.py
from pywce import HookArg, TemplateDynamicBody
def get_register_defaults(arg: HookArg) -> HookArg:
# flow requires `msisdn` field, lets set this to current user wa_id as default value
flow_defaults = {"msisdn": arg.user.wa_id}
arg.template_body = TemplateDynamicBody(initial_flow_payload=flow_defaults)
return arg
Flow Response
When user fills your flow form, the engine returns this in the additional_data
property of the hook model
A (python) hook model is as below.
Learn more about hooks in the Hooks Section
@dataclass
class HookArg:
# ...
flow: str = None
additional_data: Dict[str, Any] = None # <=== Will contain flow response data
Example on-receive
hook to process flow response.
# example/hooks.flows.py
from pywce import HookArg
from .services import UserService
def register(arg: HookArg) -> HookArg:
"""
Register user from data obtained from WhatsApp Flow
"""
print(f"Received hook arg: {arg}")
flow_auth_data = arg.additional_data
result = UserService.register(
msisdn=flow_auth_data.get('msisdn'),
email=flow_auth_data.get('email'),
password=flow_auth_data.get('pin')
)
return arg