Testing
Testing UI Package
Widgets can be tested with widget tests using flutter_test and golden tests using alchemist. Themes can be tested using basic unit tests.
Testing Platform Root Package
The package can be tested using basic unit tests.
Testing Platform Feature Package
Blocs and Cubits can be tested using bloc_test. Widgets can be tested with widget tests using flutter_test and golden tests using alchemist.
Testing Infrastructure Package
Data Transfer Objects and Service Implementations can be testet with basic unit tests.
Integration Testing (E2E)
With integration testing, developers can confidently deliver a robust user experience by verifying that all parts of the application work harmoniously together, minimizing the risk of issues arising from the integration of different functionalities and reducing the chance of unexpected problems for end-users. Every Rapid project comes with a ready-to-use integration testing setup located inside the root package of each supported platform, and supports running integration tests in multiple environments.
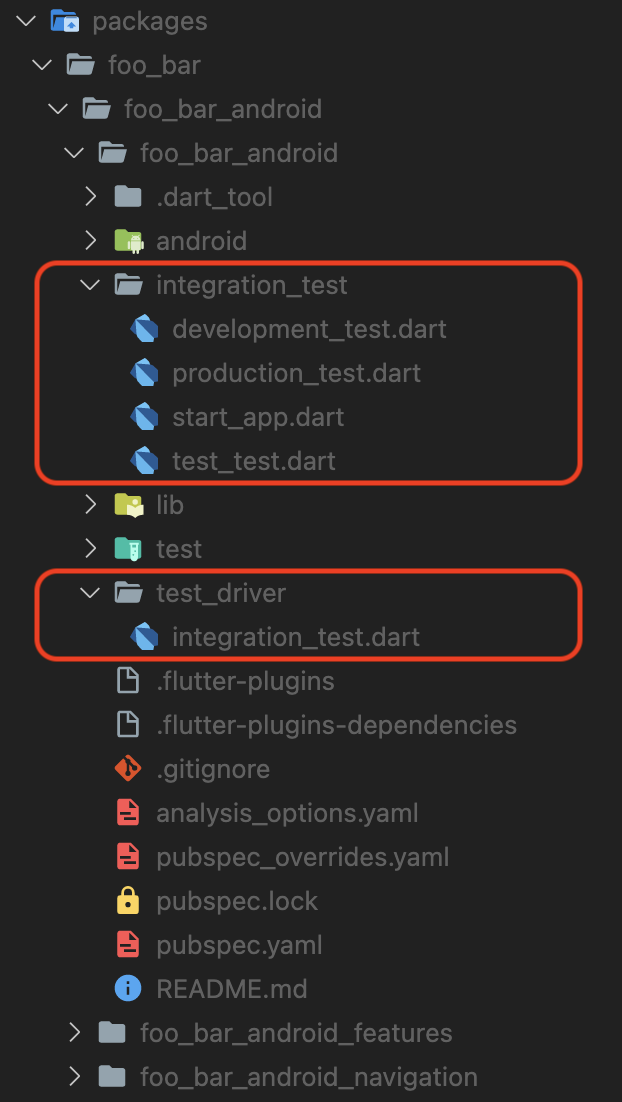
Adding Integration Tests
Adding a new integration test includes two steps:
- Create a new file inside the
integration_test
directory.
// sign_in_username_password.dart
import 'package:flutter_test/flutter_test.dart';
Future<void> performTest(WidgetTester tester) async {
await tester.pumpAndSettle();
// testing logic goes here
}
- Add the test to the environment runners.
// development_test.dart
...
import 'sign_in_username_password.dart' as sign_in_username_password;
void main() {
...
group('E2E (development)', () {
...
testWidgets('sign in with username and password', (tester) async {
await dev.main();
await sign_in_username_password.performTest(tester);
});
});
}
Running Integration Tests
# Run e2e tests in development environment
melos test:e2e:dev
# Run e2e tests in test environment
melos test:e2e:test
# Run e2e tests in production environment
melos test:e2e:prod
Web:
chromedriver --port=4444
# Run e2e tests in development environment
melos test:e2e:dev:web
# Run e2e tests in test environment
melos test:e2e:test:web
# Run e2e tests in production environment
melos test:e2e:prod:web